This database is an architecture component of Android Jetpack. Jetpack is a collection of software components / libraries which helps you to make android apps easier than you thought.
Few things that you should know about what Jetpack really does:
- Follow best practices.
- Free you from writing boilerplate code.
- Simplify complex tasks, so you can focus on the code which you care about.
It’s time to know about Room
As we know, this is a part of Android Jetpack. Room is generally another best practice like SQLite. It is a better approach in the sense of data persistence compared to SQLite.
It provides an abstraction layer over SQLite to allow fluent database access while harnessing the full power of SQLite.
This makes it easier to play around with SQLiteDatabase objects in your app. It verifies the SQL queries at compile time and removes or decreases the amount of boilerplate code.
Why not SQLite?
Each Query and Entity are checked at compile time which may prevent crash of application at runtime. A lot of boilerplate code is generated in SQLite when compared with Room Database.
We don't need to worry about writing exact queries when using room. We can just add some annotations to make Room handle itself at runtime without making an application crash.
Room is easily integrated with some of the Architecture components like LiveData. However, SQLite won't do this stuff.
We need to use lots of boilerplate code to convert between SQL queries and Java data objects in SQLite.
Components of Room Database
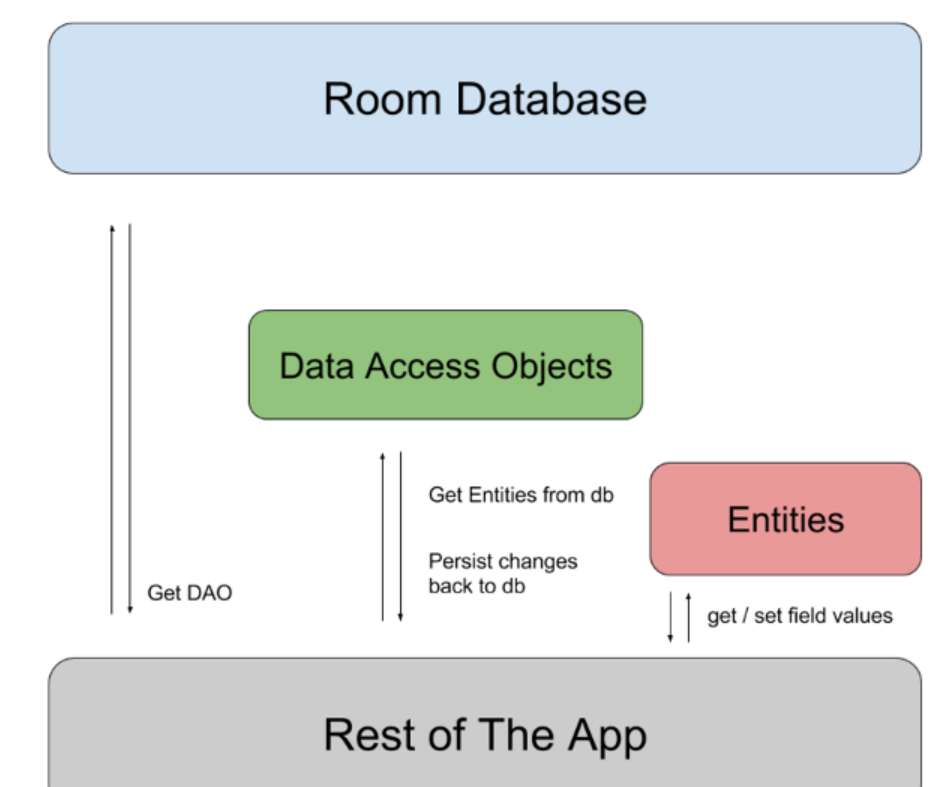
It has three components of Room Database:
1.)Entity
2.)DAO (Data Access Objects)
3.)Database
Add the dependencies
First things First, If you want to use Room in your project then add the below dependencies.
dependencies { def room_version = "2.2.5" implementation "androidx.room:room-runtime:$room_version" annotationProcessor "androidx.room:room-compiler:$room_version" implementation "androidx.room:room-ktx:$room_version" implementation "androidx.room:room-rxjava2:$room_version" implementation "androidx.room:room-guava:$room_version" testImplementation "androidx.room:room-testing:$room_version" }
Entity Class
Create an Entity class where we can define our table name and column names. This Entity class is like a data model(get & set methods). We need to create it with a number of columns we assure to take.
@Entity(tableName = "UsersDemo") public class User { @PrimaryKey public int uid; @ColumnInfo(name = "first_name") public String firstName; @ColumnInfo(name = "last_name") public String lastName; }
Here you can create it by giving @Entity annotation to declare that this is an entity class with declared information about the table.
@PrimaryKey defines that, this column is going to be the head of all the remaining columns.
@ColumnInfo is finally known to everyone. It is to show the information about each column in a table.
If we want our one of the columns to remain ignored when passing values, then we need to assign an annotation called @Ignore. This will allow Room to indicate that this column might not be important when passing values.
Note : We need to create a constructor for Entity class.
DAO (Data Access Objects)
@Dao public interface UserDao { @Query("SELECT * FROM user") ListgetAll(); @Query("SELECT * FROM user WHERE uid IN (:userIds)") List loadAllByIds(int[] userIds); @Query("SELECT * FROM user WHERE first_name LIKE :first AND " + "last_name LIKE :last LIMIT 1") User findByName(String first, String last); @Insert void insertAll(User... users); @Delete void delete(User user); }
This is used for querying. We can write all our queries inside this class. This is actually an interface which helps us to connect over Room Database.
We can directly apply annotations to this class in order to know what query is executing exactly.
This is responsible for defining the methods that access the database. Remember that, for creating DAO we need to create an interface and annotate it with @Dao.
Database
Create a custom class for the database where we can access the interface where we wrote the SQL queries with some annotations.
Create database with @Database annotation.
@Database(entities = {User.class}, version = 1) public abstract class AppDatabase extends RoomDatabase { public abstract UserDao userDao(); }
You get an instance of a created database using the above given code.
AppDatabase db = Room.databaseBuilder(getApplicationContext(), AppDatabase.class, "database-name").build();
Developers need to call each method in the DAO interface wherever you want to. To make sure that each method gets called in an activity with proper indication.